Building Fast Apps for Slow Environments
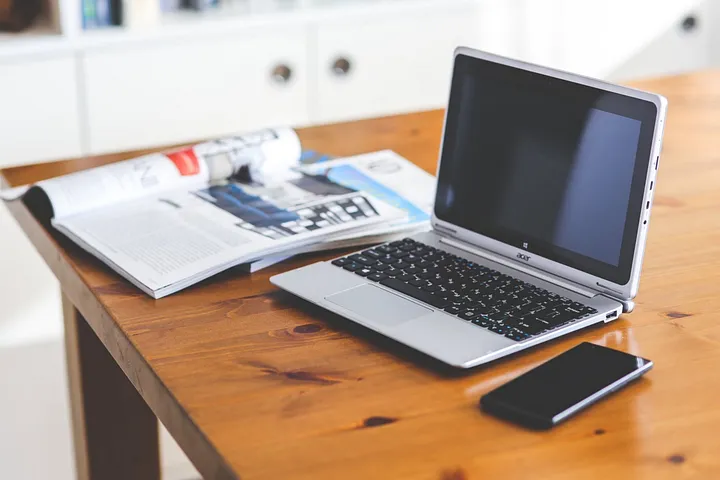
Today we are all blessed with technology. Gone is yesteryear of dial-up and slow connections. However, there are sometimes life-halting blips of slowness. To the frenzied mind, this is unacceptable. Having an app still feel fast even in times of slowness—such as not enough RAM, full disk space, or slow internet—is essential in building something people want to use.
Middleware
For every request to the database or backend, have a slowness timeout variable that you can put before every request. This will make every request take a certain amount of time. How does your website feel and load when it is slow? Back in the day—and people still do it—use technology that is blocking and make the whole thing load before even sending anything back to the browser.
Lazy Loading
Lazy loading is a design pattern that defers the initialization of an object until it's needed. In web development, this means loading content or resources only when they are required, rather than all at once. Implementing lazy loading can significantly improve the performance of your application, especially in slow environments.
- Images and Videos: Load media content only when it's in the viewport.
- Code Splitting: Break down your JavaScript bundles to load only the necessary code for the current page.
- Third-party Scripts: Delay loading analytics or advertising scripts until after the main content has loaded.
Simulating Slow Network Conditions
To effectively test how your application performs under slow network conditions, you need to simulate those environments.
- Browser Throttling: Use developer tools in browsers like Chrome or Firefox to throttle network speed.
- Network Link Conditioner: For macOS users, this tool simulates various network conditions.
- Third-party Tools: Use tools like Charles Proxy or Fiddler to introduce latency and bandwidth limits.
Testing with Limited Resources
Ensure your application runs smoothly even when system resources are constrained.
- CPU Throttling: Use browser developer tools to simulate slower CPUs.
- Memory Constraints: Test your app's performance when system memory is low.
- Disk Space Limitations: Simulate environments with minimal disk space to see how your app handles storage limitations.
Handling Full Disk Space
Applications can behave unpredictably when disk space runs out.
- Graceful Degradation: Ensure your app continues to function with reduced features.
- User Notifications: Inform users when they're running low on disk space.
- Error Handling: Implement robust error handling for write operations.
Optimizing for Performance
Performance optimization is crucial for a good user experience in slow environments.
- Minification and Compression: Reduce the size of your CSS, JavaScript, and HTML files.
- Caching: Utilize browser caching to store static resources locally.
- Content Delivery Networks (CDNs): Serve your assets from locations closer to your users.
Using Performance Profiling Tools
Identify bottlenecks in your application using profiling tools.
- Lighthouse: An open-source tool from Google for auditing performance.
- WebPageTest: Provides detailed insights into your website's load performance.
- Chrome DevTools Performance Panel: Analyze runtime performance in Chrome.
Progressive Enhancement
Build your application to provide basic functionality to all users, regardless of their environment.
- Core Content First: Ensure that the main content loads before any enhancements.
- Feature Detection: Use JavaScript to detect if advanced features are supported and load them conditionally.
- Accessibility: Make your app usable without relying on JavaScript or CSS.
Graceful Degradation
Allow your application to scale back its functionality in less capable environments.
- Fallbacks: Provide simpler alternatives for advanced features.
- Error Messages: Display meaningful messages when certain features aren't available.
- Testing Older Browsers: Ensure compatibility with older browsers that may lack modern features.
User Experience Considerations
A smooth user experience keeps users engaged, even when performance is suboptimal.
- Loading Indicators: Use spinners or progress bars to show that the app is working.
- Responsive Design: Ensure your app adapts to different screen sizes and orientations.
- Simplify Navigation: Make it easy for users to find what they're looking for quickly.
Timeouts and Retries
Network requests can fail or take too long in slow environments.
- Set Appropriate Timeouts: Prevent your app from waiting indefinitely for a response.
- Retry Logic: Implement retries with exponential backoff for transient failures.
- Offline Support: Cache data locally to allow the app to function without a network connection.
Error Handling
Robust error handling improves reliability.
- Catch Exceptions: Prevent crashes by handling unexpected errors gracefully.
- User Feedback: Inform users of issues and provide actionable steps.
- Logging: Implement logging to capture errors for future analysis.
Asynchronous Operations
Keep your app responsive by offloading heavy tasks.
- Web Workers: Run scripts in background threads.
- Async/Await: Simplify asynchronous code for better readability.
- Non-blocking UI: Ensure that the user interface remains responsive during background operations.
Performance Budgeting
Set performance goals to keep your app lean.
- Resource Limits: Define maximum sizes for assets like images and scripts.
- Monitor Metrics: Regularly check load times, time to interactive, and other key performance indicators.
- Automated Testing: Integrate performance tests into your CI/CD pipeline.
Conclusion
Testing in slow environments is essential for building resilient applications that offer a great user experience under all conditions. By simulating slow networks, optimizing performance, and handling errors gracefully, you ensure that your app remains usable and efficient, keeping your users satisfied even when technology fails them.